Hi! I am Bartosz Sekuła
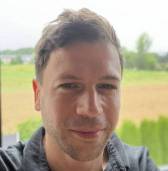
Over the past 18 years I have worked in both consulting and product development. At heart I am a passionate developer, I love to learn new things and write code.
Since 2016 I've been working in Optimizely as a fullstack developer.
Additionally, I am one of the creators and maintainer of a few popular add-ons like:
- Advanced Reviews - an Open Source add-on that improves the reviewing process and lets external users to view & review content items or whole projects without the need to access the Edit Mode
- Block Enhancements - very popular add-on which introduced commands like Quick Edit, Draft Mode and similar
- Content Manager & Grid View - an addon which provides an alternative editing UI so that it's not needed to go to the default Edit Mode