Apparently dojo has issues with their localization modules that makes it impossible to determine the correct culture info when you have two languages from the same locale registered at the same time.
For example if your editors are from let's say New Zealand the because of this dojo bug https://bugs.dojotoolkit.org/ticket/17155 the language formatting would always fallback to the _ROOT_ nls for them (which is "en" in this case).
It's a very annoying issue as for example number and datetime formatting are different for English editors from US and from GB/NZ/AU.
One could try to solve it by changing the globalization of the whole site or by turning languages in admin mode on and off but in fact the Edit Mode is the only place where we need the change to be visible in.
If you add this simple initializable module to your site it would just affect dojo i18n module without touching the asp.net runtime.
using System;
using EPiServer.Framework;
using EPiServer.Framework.Initialization;
using EPiServer.Shell.UI;
namespace AlloyMvcTemplates
{
[InitializableModule]
[ModuleDependency(typeof(EPiServer.Web.InitializationModule))]
internal class DateFormatModifier : IInitializableModule
{
private void DojoConfigOnSerializing(object sender, EventArgs eventArgs)
{
var config = (DojoConfig) sender;
if (config.Locale.StartsWith("en"))
{
config.Locale = "en-gb";
}
}
public void Initialize(InitializationEngine context)
{
DojoConfig.Serializing += DojoConfigOnSerializing;
}
public void Uninitialize(InitializationEngine context)
{
DojoConfig.Serializing -= DojoConfigOnSerializing;
}
}
}
The example above will make sure that all your English editors will always use the "en-gb" language tag so for example date/times would no longer be en-us formatted (default English formatting).
You won't have to change anything about the available locales in the My Settings screen, users can just select English and it would automatically use en-gb instead of the default en-us.
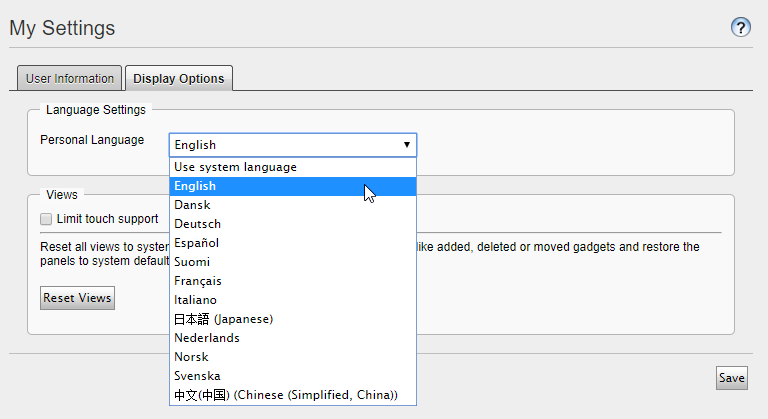
If you use the Forms addon then you will also have to do a little trick as the dgrid datetime formatter is set to a fixed pattern 'yyyy-MM-dd'
In order to change it please add the following module:
define([
"dojo/_base/declare",
"dojo/date/locale",
"epi-forms/dgrid/Formatters"
], function (
declare,
locale,
Formatters
) {
return declare([], {
initialize: function () {
Formatters.dateTimeFormatter = function (value) {
return locale.format(new Date(value), { datePattern: "dd-MM-yyyy", timePattern: "HH:mm:ss" });
};
}
});
});
And register it in your module.config
<clientModule initializer="alloy/formatters-initializer">
<moduleDependencies>
<add dependency="EPiServer.Forms.UI" type="RunAfter" />
</moduleDependencies>
</clientModule>
That way you will also get a custom date formatting in the "Form submisstions" dgrid:
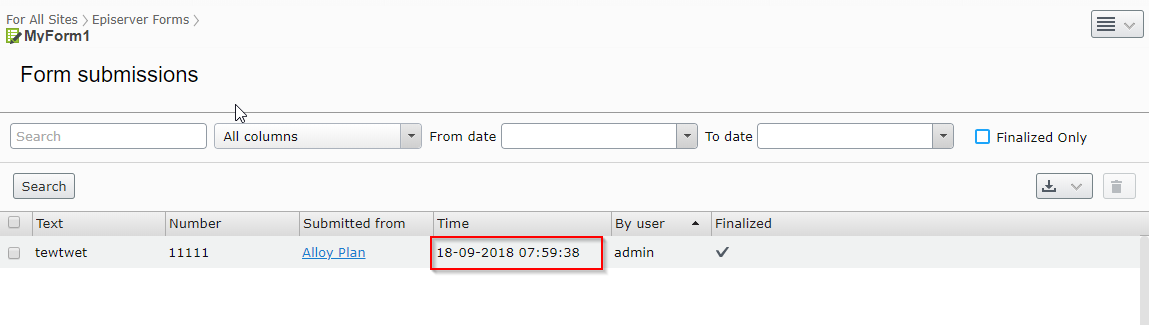